OpenCV - Getting Started
Images
What are Images?
- 2-Dimensional representation of the visble light spectrum
- Each pixel point corresponds to a different color which means that reflects different wavelengths of light
How are images formed?
- When light reflects off an object at different points and bounces off onto a film, sensor or retina
- Essentially use a barrier to block off most points of light while leaving a small gap (aperture)
- Aperture allows some points of light to be reflected onto the film
A simple pinhole camera model (Above image) and the aperture is always fixed. So that means that a constant amount of light is always entering this hall which can be sometimes overpowering for the film. Meaning that everything is going to look white.
Therefore It need to be fixed. See below explaination!
Controlling Image Formation with a Lens
Both our eyes and camera use an adaptive lens to control many aspects of image formation:
- Aperture Size
- Controls the amount of light allowed through ( f-stops in cameras)
- Depth of Field (Bokeh)
- Lens width
- Adjust focus distance (near of far)
How Human see images?
The human visual system (eye & visual cortex) is incredibly good at image processing.
They’re remarkably good at focusing quickly seeing in varying light conditions and picking up sharp details and then in terms of it to printing what we see humans are exceptional at this as we can quickly understand the context of different images and quickly identify objects faces you name it we can actually do this far better than any computer vision technique right now.
Our brains do this by using six layers of visual processing.
How do Computer store images?
- Computer use RGB color space by default. What is RGB?
- Each pixel corrdinate (x,y) of a 2D plane contains 3 values ranging for intensities of 0 to 255 (8-bit).
- Red
- Green
- Blue
Images are stored in multi-dimensional arrays
1-dimensional array
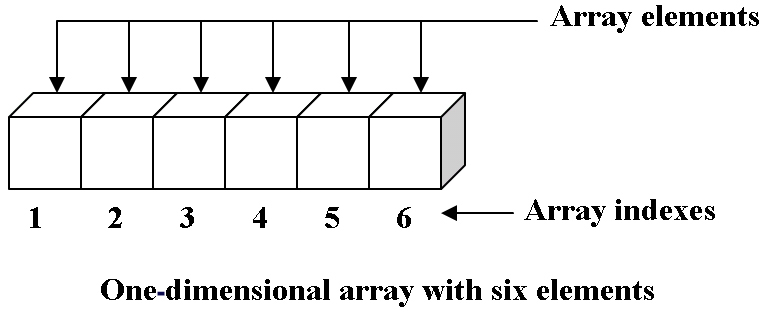
2-dimensional array
Think of it like an x y coordinate system.
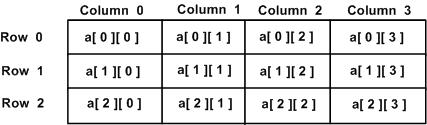
3-dimensional array <- Where images are stored
Think of this as many two dimensional arrays stacked together.
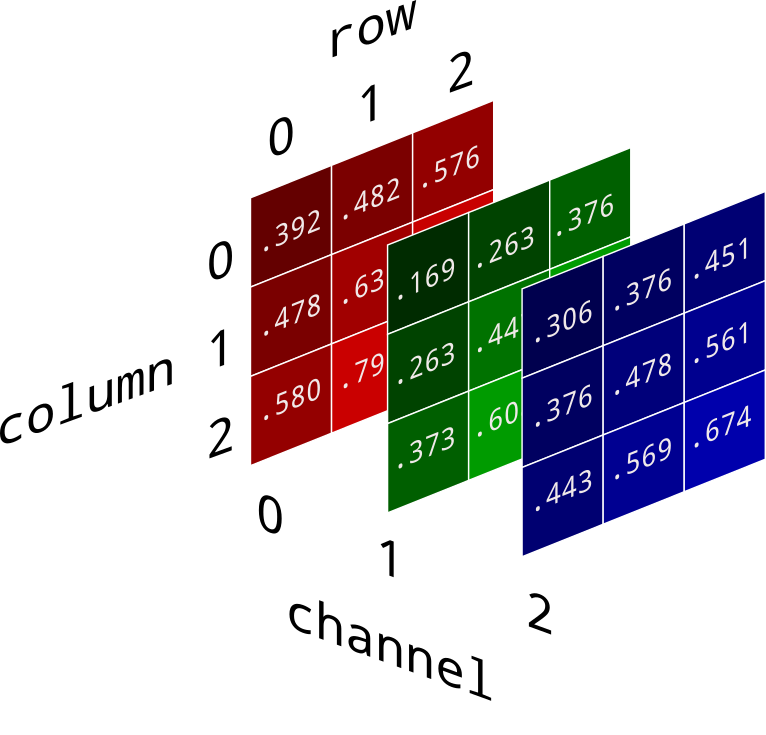
Black and White or Greyscale
- Black and White images are stored in 2-Dimensional arrays.
- Two Types of B&W images :
- Grayscale - Ranges of shades of grey (255 - 0)
- Binary - Pixels are either black or white (255 or 0)
OpenCV
What is OpenCV?
OpenCV (Open Source Computer Vision) is a library of programming functions mainly aimed at real-time computer vision. Originally developed by Intel, it was later supported by Willow Garage then Itseez. The library is cross-platform and free for use under the open-source BSD license.
You can use either C++ or Python with it.
OpenCV in Python
Why Python?
- Python allows us to do to easily grasp complex concepts.
- Python is one of the easiest easiest languages for beginners
- It is extremely powerful for data science and machine learning applications
- It stores images in numpy arrays which allows us to do some very powerful operations quite easily
Checking my OpenCV version
1 | # Import OpenCV |
Loading and Displaying a Image
1 | # Import OpenCV |
ok, lets try the code
1 | import cv2 |
Getting Info of the Image
To take a closer look at how images are stored, we need to use numpy
.
1 | # Import numpy |
Save Images We Edit in OpenCV
1 | # Use 'imwrite' specificing the file name and the image to be saved (generated) |
Which IDE should I use?
Normal IDEs
1 | import cv2 |
I prefer Jupyter Notebook.
The Jupyter Notebook is a living online notebook, letting faculty and students weave together computational information (code, data, statistics) with narrative, multimedia, and graphs. Faculty can use it to set up interactive textbooks, full of explanations and examples which students can test out right from their browsers. Students can use it to explain their reasoning, show their work, and draw connections between their classwork and the world outside. Scientists, journalists, and researchers can use it to open up their data, share the stories behind their computations, and enable future collaboration and innovation.
Jupyter Environment
Grayscaling
Grayscaling is process by which an image is converted from a full color to shades of grey.
What is Grayscaling in OpenCV?
Grayscale is a range of shades of gray without apparent color. The darkest possible shade is black, which is the total absence of transmitted or reflected light. The lightest possible shade is white, the total transmission or reflection of light at all visible wavelengths.
In OpenCV many functions grayscale images before processing. We use grayscaled images because it simplifies the image (something like noise reduction) and this would increase the processing time of our program as there is less information in the image. Color information is a bonus but it’s not all that necessary.
Convert Color Image into Grayscale
1 | import cv2 |
Short Cut Converting Image into Grayscale
1 | # faster method |
Now the output should be like this :
Color Science
There are many Standards of Colors.
RGB Color Space
RGB is an additive color model that generates colors by combining blue, green and red and different intensities/brightness.
OpenCV’s default color space is RGB.
RGB Color Model

- Each colour appears in its primary spectral components of R, G and B
- A 3-D Cartesian co-ordinate system
- All axis are normalized to the range of 0 to 1
- The Grayscale (Points of equal RGBB values) extends from origin to farthest point
- Different colors are points on or inside the cube
- Images comprise 3 independent image plances (each for R,G,B respectively.)
- The number of bits representing each pixel in RGB space is called pixel depth
- Each R,G,B image is an 8-bit image
- Each RGB color pixel has a depth of 24 bits - full-color image.
- The total number of colors =

Note : OpenCV actually stores color in the BGR format.
Why? We use BRG order on computers due to how unsigned 32-bit integers are stored in memory, it still ends up being stored as RGB. The integer representing a color e.g. 0x00BBGGRR will be stored as 0xRRGGBB00.
CMYK Color Space
CMY Model
CMY means Cyan, Magenta and Yellow.
- Secondary Colors of light or primary colors of pigments
- Model for printers (color pigments on paper)
CMYK Model
Sometimes, a CMYK model (K stands for Black) is used in color printing to produce darker black.

^ CMY and CMYK is not really related to OpenCV. Just for your information.
HSV Color Space
HSV (Hue, Saturation & Value/Brightness) is a color space that attempts to represent colors the way human perceive it. It stores color information in a cyclindrical representation of RGB color points.
HSV Color Model
- Hue - Color Value (0-179)
- Saturation - Vibrancy of Color (0-255)
- Value - Brightness or Intensity (0-255)
HSV is useful in computer vision for color segmentation. In RGB, filtering specific colors isn’t easy. However, HSV makes it much easier to set color ranges to filter specific colors as we perceive them.
Color Filtering
The Hue (Hue color range, goes from 0 to 180. NOT 360) and is mapped differently than standard in OpenCV.
Color Range Filters:
- Red - 165 to 15
- Green - 45 to 75
- Blue - 90 to 120
Why is the range of Hue 0-180° in OpenCV?
Now you understand why I said Hue is 0-179 in HSV space. 180 = 0.
Color Space
How Color Space affect the color levels
Note OpenCV’s RGB is not RGB but BGR.
1 | import cv2 |
Now lets convert it to grayscale.
1 | # Convert to grayscale |
Convert Image into HSV color space
1 | import cv2 |
The HSV image looks quite cool.
Individual Channels in an RGB Image
Amplifying
1 | import cv2 |
Only One Color
1 | import cv2 |
1 | print(image.shape[:2]) |
Histogram
Histogram are a great way to visualize individual color components of Images
Import Matplotlib
To Create Histogram we need to import the matplotlib.
1 | from matplotlib import pyplot as plt |
Function cv2.calcHist()
We will use a function cv2.calcHist()
.
cv2.calcHist(images,channels,mask,histSize,ranges[, hist[, accumulate]] )
- images - source image, given in square brackets
- channels -
[0]
for grayscale,[0]
or[1]
or[2]
for color image (blue, green or red channel)- mask - for finding particular region of image
- histSize -
[256]
for full scale- ranges - Normally
[0, 256]
1 | image = cv2.imread('./images/Z.jpg') |
Plot it out
Now let’s plot it out.
1 | # we plot a historgram, `ravel()` flatens our image array |
Full Code
1 | import cv2 |
Output :

Drawing
You can use OpenCV to draw images and shapes.
To Plot the Image, use
plt.imshow(image)
.Note
plt.imshow(image)
usesRGB
instead ofBGR
.You might want to use
cv2.cvtcolor(img,cv2.COLOR_BGR2RGB)
to change it back to RGB for ploting.For photos, you can use plot for quicker access.
Drawing a Square
1 | # Create a black color image |
Drawing a Line
cv2.line(image,starting cordinates, ending cordinates, color, thickness)
- image - your image to draw on
- starting cordinates - (x,y)
- ending cordinates - (x,y)
- color - (Blue value, Green value, Red value)
- thinkness - measured in pixel
1 | # Create a black color image |
Drawing a Rectangle
cv2.rectangle(image,starting vertex, opposite vertex, color, thickness)
1 | # Draw a Rectangle in |
Drawing a Circle
cv2.circle(image, center, radius, color, fill)
1 | image = np.zeros((512, 512, 3), np.uint8) |
Drawing Polygons
1 | image = np.zeros((512,512,3), np.uint8) |
Adding Text
cv2.putText(image, text, starting point, font, font size, color, thickness)
- image - your image to draw on
- text - The text you want to display
- starting point - (x,y) from botton left
- font
cv2.FONT_HERSHEY_SIMPLEX or
cv2.FONT_HERSHEY_PLAIN`cv2.FONT_HERSHEY_DUPLEX or
cv2.FONT_HERSHEY_COMPLEX`cv2.FONT_HERSHEY_TRIPLEX or
cv2.FONT_HERSHEY_COMPLEX_SMALL`cv2.FONT_HERSHEY_SCRIPT_SIMPLEX
cv2.FONT_HERSHEY_SCRIPT_COMPLEX
- font size - measured in pixel
- color - (Blue value, Green value, Red value)
- thinkness - measured in pixel
1 | image = np.zeros((512,512,3), np.uint8) |
Reference
Installation of OpenCV using Anaconda MacOS (conda / pip approach)
Install OpenCV 4 on macOS (brew approach)
R.C. Gonzalez and R.E. Woods, Digital image processing (4th ed.) New York, NY: Pearson 2018.